Data Fetching best practices
Data fetching is a critical aspect of web application performance. While Alokai leverages established best practices from frameworks like Next.js and Nuxt, this guide explains how to effectively implement these strategies in the context of Alokai's architecture.
Read about data fetching in general in Next.js / Nuxt official documentation:
Data fetching strategies
There are three data-fetching strategies:
- Client-Side Fetching: Data is fetched by the client (usually a browser) directly from an API.
- Server-Side Fetching / Server-Side Rendering (SSR): Data is fetched on the server during the request and delivered to the client as pre-rendered HTML.
- Static Site Generation (SSG): Pages are pre-rendered at build time, suitable for content that doesn't change frequently. E-commerce applications are highly dynamic, so we’re not covering this strategy as it’s rarely used in this context. Cached server-side rendered pages are a good replacement for SSG.
The diagram helps to visualize the two first strategies:
The Storefront
is a Next/Nuxt application. Api is any 3rd party system.
Animation below visualizes the difference between server and client side fetching. Product data is fetched on the server and rendered to HTML, so the product data is visible immediately after page load. Cart data is fetched client side, so we see a spinner that is replaced by content when cart data is loaded.
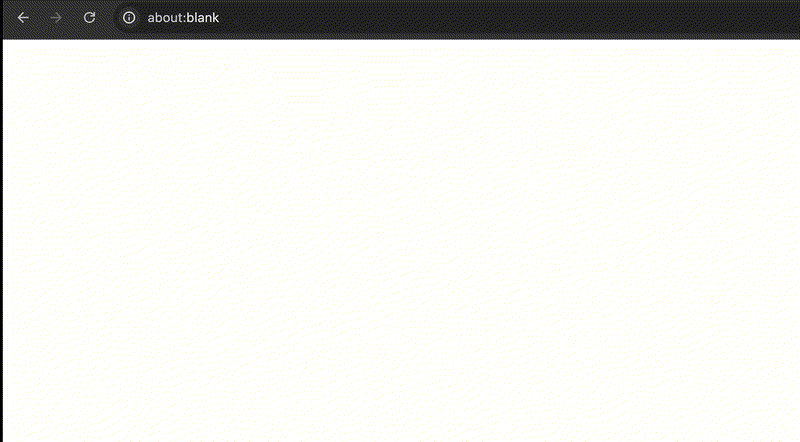
How to pick data fetching strategy
The rule of thumb is: always use server-side fetching except for:
- lazy loaded data - data that is not visible immediately on page load and can be removed from server-side rendered html to reduce its size. For example:
- product reviews should appear only when user clicks expand on the “reviews accordion”
- content below the fold is fetched on scroll
- personalized data - data that is different for each user or group. Fetching such data server side would not only cause flickering but also might cause problem with caching (read more about caching). For example:
- pricing data might be different for each customer group
- product recommendations are based on individual user’s journey
- cart content is specific for each user
Data fetching in Alokai
Alokai architecture introduces the Middleware in between the front-end application and the APIs. This adds two additional possible routes to our diagram, but most of the traffic should go through the middleware, because:
- it keeps your architecture simple
- you can easily monitor middleware traffic in the console
- enables data federation
- ensures middleware reusability and omnichannel capabilities
Fortunately, we can reject the route between the server and the API, because:
- effectively it is similar to reaching through the middleware, but adds unnecessary complexity to the architecture,
- tightly couples the storefront with the API.
The direct route between the browser and API should be avoided, because it:
- tightly couples storefront with the API,
Use direct direct browser to API calls only when communicating via middleware is not possible or requires unnecessary effort. Sample scenarios when it might be valid to communicate directly between client and API:
- the communication requires extremely low latency - e.g. voice or video stream
- communication with API is done via some SDK that requires direct client (browser) interaction - e.g. analytics or authentication services
Examples
Server-Side fetching in Next.js
In this example we fetch product data on the server and display it's name.
import { getSdk } from '@/sdk';
export default async function ServerSideDataFetching() {
const sdk = getSdk(); // get the SDK instance on the server side.
const response = await sdk.unified.getProductDetails({ id: '300013358' }); // fetch product data
const product = response?.product ?? null;
return <h1>Product name: {product.name}</h1>; // display product name
}
Client-side fetching in Next.js
In this example we fetch product data on the client. We display loader while data is being fetched. Tanstack query is used to fetch data and manage component state.
'use client'; // tell nextjs to render this component on the client side
import { SfLoaderCircular } from '@storefront-ui/react';
import { useQuery } from '@tanstack/react-query';
import { useSdk } from '@/sdk/alokai-context';
export default function ClientSideDataFetching() {
const sdk = useSdk(); // get the SDK instance on the client side.
// use tanstack query to fetch product data and manage the loading state
const { data: productData, isLoading } = useQuery({
queryFn: () => sdk.unified.getProductDetails({ id: '300013358' }),
queryKey: ['product'],
});
// display a loader while product data is loading
if (isLoading || !productData) {
return <SfLoaderCircular />;
}
return <h1>Product name: {productData.product.name}</h1>; // display product name
}